Sigfox devices
SIGFOX is a connectivity solution that focuses on low throughput devices. On SIGFOX your device can send between 0 and 140 messages per day. This is a maximum of one message every 11 minutes, if you want a 24h distribution. And each message can be up to 12 bytes of actual payload data. You can also transmit 4 messages of 8 bytes payload to each device per day. In order to receive messages, the device has to request for data from your server, which means that it has to be programmed to receive data at specific events or at specific times.
The protocol already transmits the device ID, so the 12 and 8 bytes represent the actual payload and there is no limit on how you can structure the payload. SIGFOX does not understand or transform your payload, only you know how it is structured.
12 bytes easily covers the needs for devices that transmit data such as the location of a device, an energy consumption index, an alarm, or any other type of basic sensor information. The 8 bytes sent to devices allow you to send configuration data if needed, but you can optimize battery life by only being one-way if you do not need two-way communications.
How to use Sigfox (Uplink) with thethings.iO
The Sigfox backend can automatically forward some events using the «callback» system. The Callback system enables you to use a thethings.iO callback url that lets thethings.iO backend receive the Sigfox device messages. This lets you take advantage of the thethings.iO features such as analytics, cloud code, interoperability, etc.
The configuration of callbacks is done in the device type page.
The callbacks are triggered when a new device message is received or when a device communication loss has been detected.
A callback has a set of common variables:
- device: device identifier (in hexadecimal – up to 8 characters <=> 4 bytes)
- data: the user data (in hexadecimal)
- others: time, duplicate, snr, station, data, avgSnr, lat, lng, rssi and seqNumber
Next is an example of the callback configuration form with only device (id) and data (data)
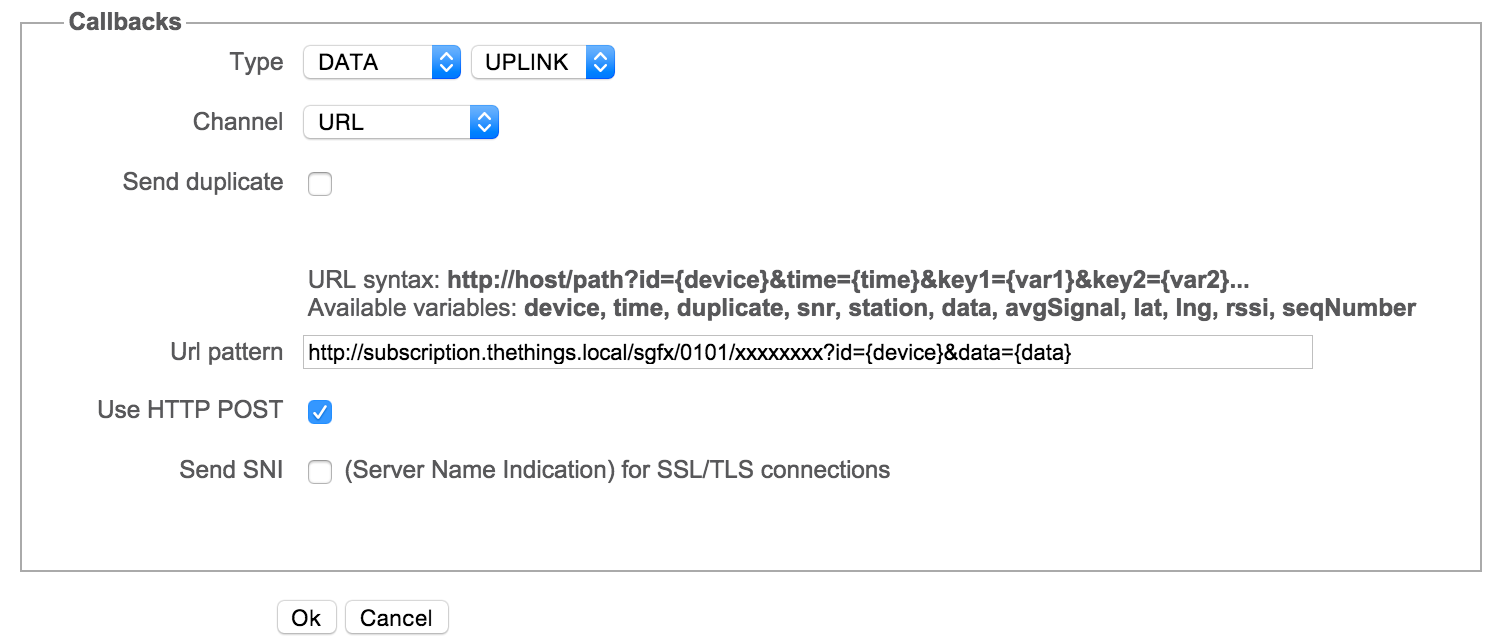
How to configure thethings.iO to use Sigfox
thethings.iO backend has to be configured to manage a Sigfox device. Follow the next steps:
Create a new product
You have to create a new product and choose Sigfox as a Serialzation Format.
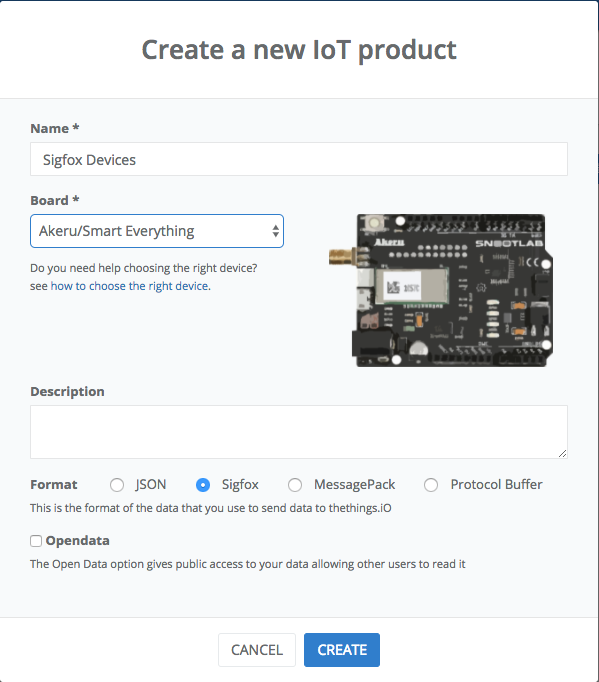
Get the subscription URL
Once you have created a Sigfox product, click on the product to get the subscription URL that you have to use as a callback URL at the sigfox backend.
This URL only gets the device and data variables. If you need other values like time, duplicate, snr, station, data, avgSnr, lat, lng, rssi and seqNumber; you have to include a key value for each one at the URL query. By default only device and data are added.
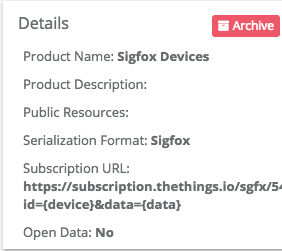
Parse the Sigfox Payload
When you create a new sigfox product, a function with the name 'sigfox_parser' is automatically created. You have to edit that function and insert the code that parses the sigfox payload. Please, don't change the name of this function. Any name other than 'sigfox_parser' will not work.
You get the Sigfox deviceId (device) and the payload (data) from the params parameter. You can access both with params.deviceId and params.data
function main(params, callback){
var result = [
{
"key": "temperature",
"value": parseInt('0x'+params.data.substring(0,2))
}
]
callback(null, result)
}
To access to the other values (time, duplicate, snr, station, data, avgSnr, lat, lng, rssi and seqNumber), you have to use: params.custom.time, params.custom.duplicate, etc.
You can also geolocate your device to be displayed on a map with the lat and lng values. You have to store this values at the $geo key.
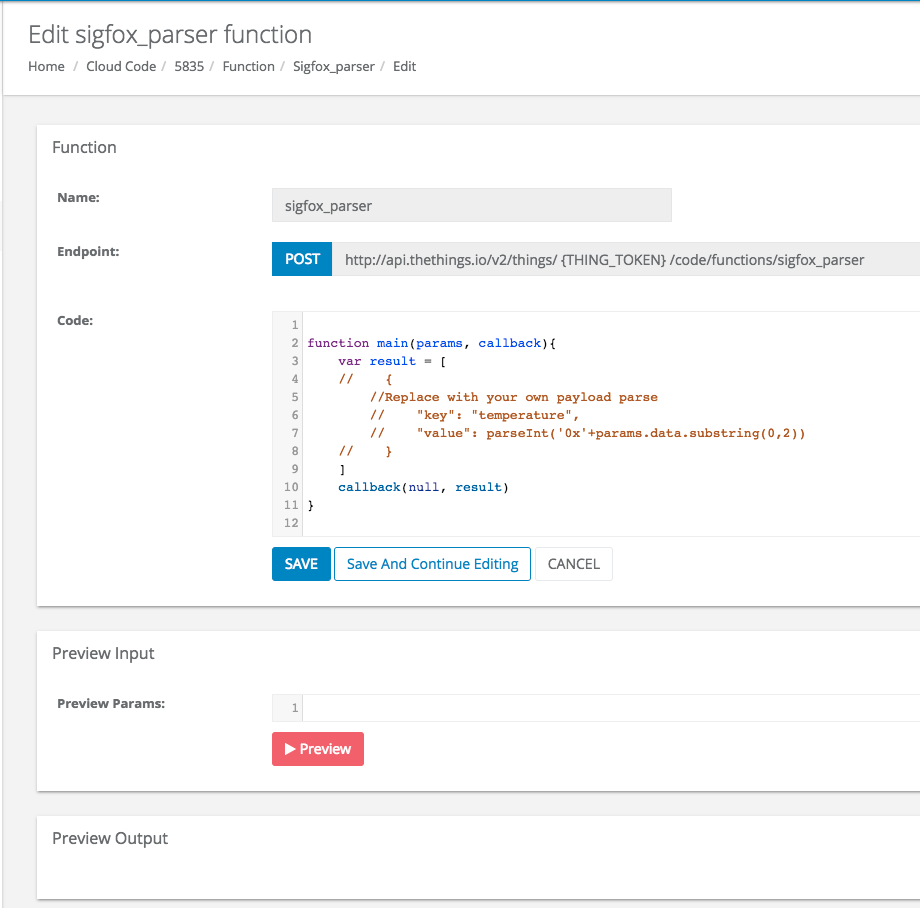
/*
You can preview this function with this preview params:
{
"deviceId": "00F2302",
"data": "20",
"custom": {
"rssi": 1.2,
"lat": 41.41,
"lng": 2.17
}
}
If you use Postman, you have to set this body:
{
"id": "00F2302",
"data": "20",
"rssi": 1.2,
"lat": 41.41,
"lng": 2.17
}
*/
function main(params, callback){
var result = [
{
"key": "temperature",
"value": parseInt('0x'+params.data.substring(0,2))
},
{
"key": "rssi",
"value": params.custom.rssi
},
{
"key": "$geo",
"value": [params.custom.lng, params.custom.lat]
}
]
callback(null, result);
}
As a result you have to return an array of objects with this structure:
[ {"key": temperature, "value": 21}, {"key": humidity, "value": 50} ... ]
Sigfox Custom Payload
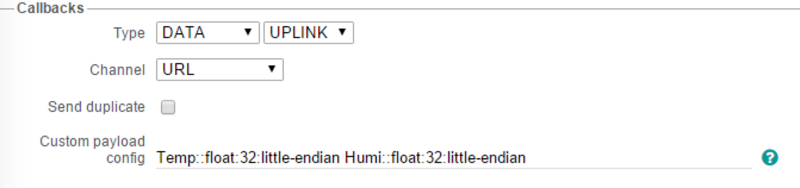
If you use the Custom payload config option, you can access that data too. At this example Temp and Humi resources are defined. To access them at the cloud code function you can do this:
var temperature = params.custom.Temp
var humidity = params.custom.Humi
Sigfox Errors
When you get a 500 Internal server error or a 600 response timeout, you have to check the next steps to find where or why is this error. Our api returns an error message if something goes wrong, but the Sigfox backend don't show this error message, only shows a 500 internal server error.
- Check if your subscription plan is expired
- Check if you have exceeded your monthly api calls rate.
- Check if you have reached your devices limit.
- Check if your sigfox_parser function exists and is working properly. Use postman to simulate a call.
- Check the resource sgfx-error of your device. There are stored some errors from the sigfox_parser code.
- Check if the data that your device sends is at the sgfx-payload resource, and this data is correct.
Updated 20 days ago