HTTP Integrations
HTTP Integrations allow you to send information with your customized body or integrating a URL to a third party service to forward the data.
Some Use Cases don't let you send the data to our platform using our REST API. For instance, lets imagine you are using a third party service, like a LORA platform that lets you forward data providing an URL. To solve this, we provide an special endpoint that lets you send the data to our platform and parse the data directly with a Cloud Code parser.
1. Get and customize your callback URL
The first step is to understand how you can customize the URL, to adapt to your specific Use Case.
Base path
The base path of the URL is: https://subscription.thethings.io/http/{deviceGroupId}/{hash}
The deviceGroupId and the hash parameters can be found at the device group details section.
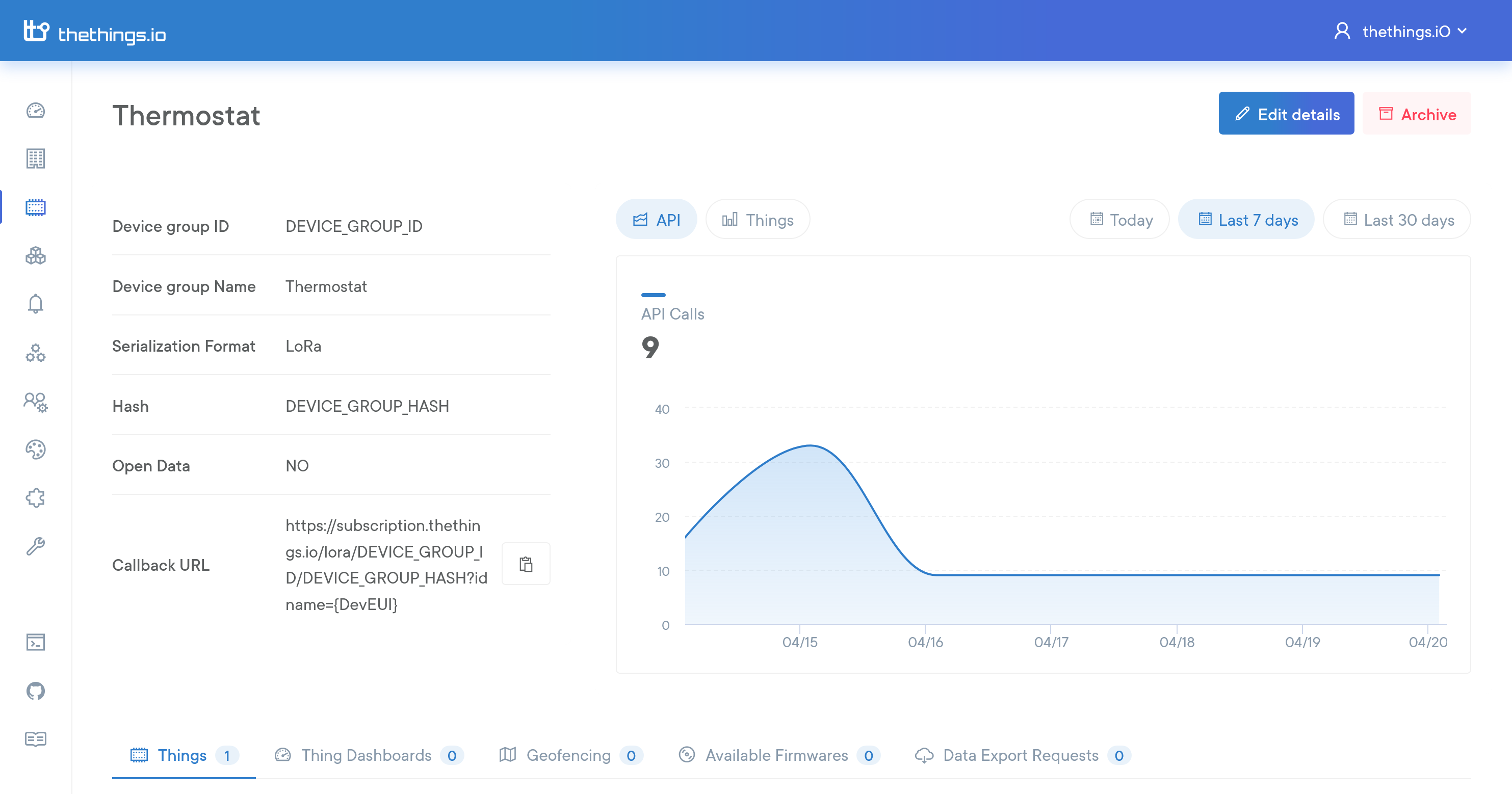
Query parameters
The query parameters lets you customize the way the integration is done. There are 3 reserved parameters: idname, thingToken and fname.
Parameter | Default | Description |
---|---|---|
idname | thingToken | Optional. Name of the key that contains de deviceId. The key could be located at the query parameters or at the payload. |
thingToken | null | Optional. If no idname is set, assumes that the key that contains the deviceId is thingToken. thingToken value is the auth token from thethings.iO |
fname | http_parser | Optional. Name of the Cloud Code parser used to parse the payload. |
You can add more query parameters. This additional query parameters will be sent as a payload to the Cloud Code parser function.
// The most simple call. Assumes that deviceId is a thingToken key from the
// body and the Cloud Code parser to call is http_parser
POST https://subscription.thethings.io/http/0000/LwwcBxzoaj
{"thingToken":"ffiwo22dsfwnlewfew22", "temperature": 10}
// The most simple way to store data to a thing. Specifies the thingToken and
// sends the temperature value to the Cloud Code parser http_parser
GET/POST https://subscription.thethings.io/http/0000/LwwcBxzoaj?thingToken=hgosnklG&temperature=10
// Specifies the body deviceId key, and parser name.
POST https://subscription.thethings.io/http/0000/LwwcBxzoaj?idname=dev_id&fname=parser
{"dev_id":"Fe34Maa2", "temperature": 10}
// Specifies the deviceId key, and parser name and all the keys are
// query parameters
GET/POST https://subscription.thethings.io/http/0000/LwwcBxzoaj?idname=dev_id&dev_id=Fe34Maa2&fname=parser&temperature=10
2. Body payload
All the query parameters (idname, thingToken and fname) can be defined as a key, value at the body payload. All the body data will be sent as a payload to the Cloud Code parser function.
// The most simple body. Assumes that deviceId is a thingToken key from the
// body and the Cloud Code parser to call is http_parser
POST https://subscription.thethings.io/http/0000/LwwcBxzoaj
{"thingToken":"ffiwo22dsfwnlewfew22", "data": {"temperature":10, "humidity":10}}
// All query parameters placed at the body
POST https://subscription.thethings.io/http/0000/LwwcBxzoaj
{"idname":"dev_id","dev_id":"ffiwo22dsfwnlewfew22", "fname":"parser", "data": {"temperature":10, "humidity":10}}
If you can't modify the body, because you are using a third party provider, you can configure the query parameters as explained at the previous section. You only have to define at the query parameters which property of the body contains the id of the device, or provide this id at the query parameters too.
3. Cloud Code parser
The last step is to use our Cloud Code service to code a javascript function to parse the payload sent by your devices.
You have to create a parser that matches the name that you have set at the fname parameter. If it's NOT set, the default name is 'http_parser'.
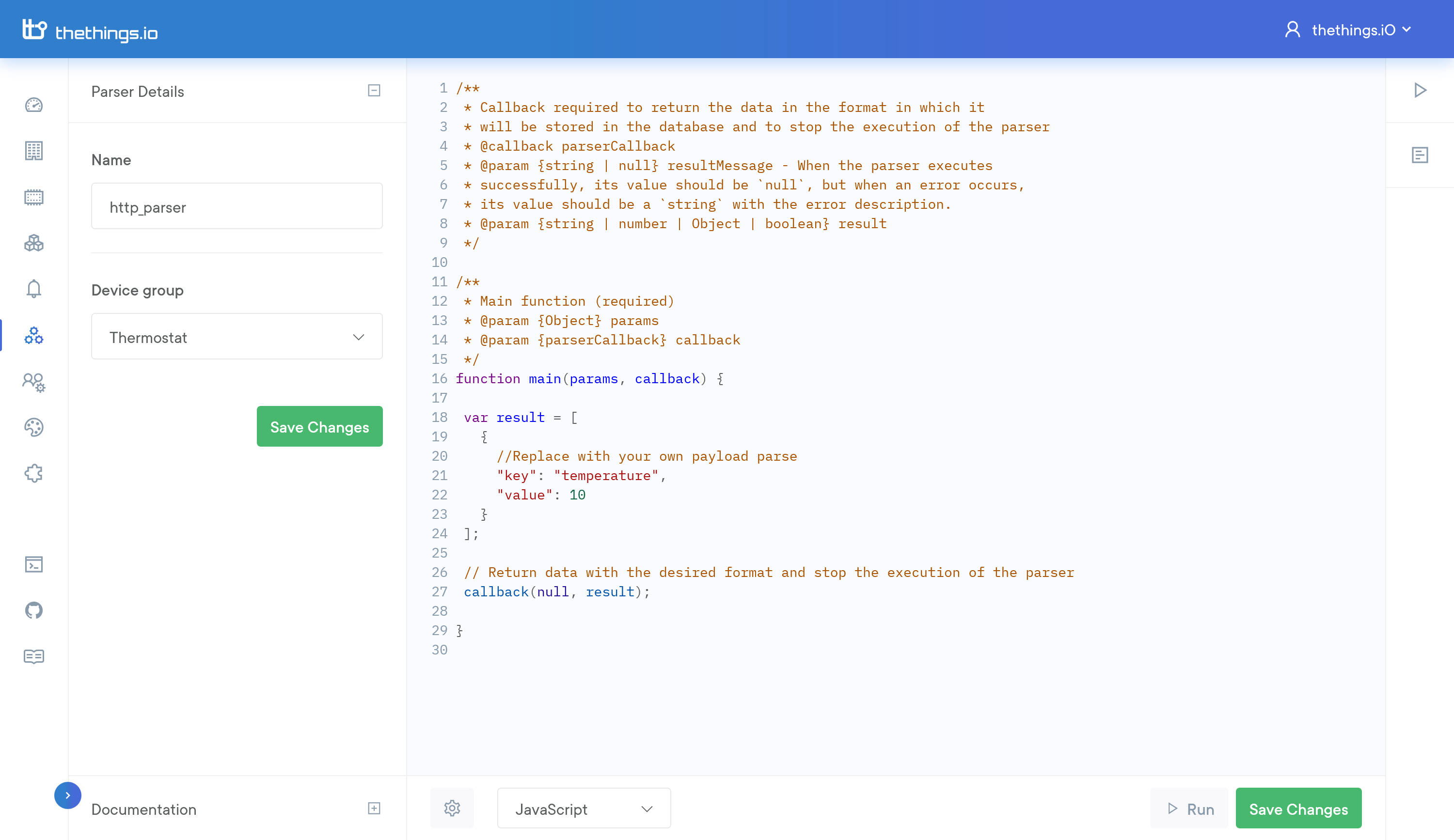
This parser has two parameters, params and callback. The params, contains all the data from the body and the query parameters. And callback, it's used to sent the result of the parser function into our platform and store the values with our JSON formatting.
/*
// All query parameters placed at the body
POST https://subscription.thethings.io/http/0000/LwwcBxzoaj
{
"idname":"dev_id",
"dev_id":"ffiwo22dsfwnlewfew22",
"fname":"parser",
"data": {"temperature":10, "humidity":10}
}
*/
/*
params = {
thingToken: thingToken,
deviceId: deviceId,
payload: {
"idname":"dev_id",
"dev_id":"ffiwo22dsfwnlewfew22",
"fname":"parser",
"data": {"temperature":10, "humidity":10}
}
};
*/
function main(params, callback){
var result = [
{
"key": "temperature",
"value": params.payload.data.temperature
},
{
"key": "humidity",
"value": params.payload.data.temperature
},
{
"key": "$settings.name",
"value": params.payload.dev_id //sets de dev_id as a name of the thing.
}
]
callback(null, result);
}
As a result you have to return an array of objects with this structure:
[ {"key": temperature, "value": 21}, {"key": humidity, "value": 50} ... ]
If the execution of the parser works, you'll find some resources created automatically at your device details: ctm_payload and ctm_error.
Errors
Our api returns an error message if something goes wrong, but you could also check:
- Check if your subscription plan is expired
- Check if you have exceeded your monthly api calls rate.
- Check if you have reached your devices limit.
- Check if your parser function exists and is working properly. Use postman to simulate a call.
- Check the resource ctm_error of your device. There you will find errors from the parser code.
- Check if the data that your device sends is at the ctm_payload resource, and this data is correct.
A. Integration example with The Things Network
With HTTP Integrations it's easy to configure a URL to send data from other data sources, a 3rd party providers. As an example, we will show how to get data from The Things Network thanks to their own HTTP Integration service: https://www.thethingsnetwork.org/docs/applications/http/.
The Things Network use a technology called LoRaWAN to provide data connectivity, it allows for things to talk to the internet without 3G or WiFi. So no WiFi codes and no mobile subscriptions. It also features low battery usage, long range and low bandwidth. Perfect for the internet of things.
The Things Network HTTP Integration service let's you set an URL to send uplink data, but you can't modify the body that it's sent. No problem, our URL is enough flexible to let you configure the uplink service.
First of all, you have to configure the url. Place the device group Id and the hash as explained at the section Get and customize your callback URL. You also need to configure the query params like this: ?idname=dev_id This tell to thethings.iO where to find the device Id at the body that The Things Networks sends to us.
The resulting URL could be like this:
https://subscription.thethings.io/http/0000/pppppptttttt?idname=dev_id
Insert this url at the HTTP Integration section of The Things Network Platform.
Then, go to thethings.iO Cloud Code section and create a new parser with the name http_parser. The body sent by The Things Network is accesible at params.payload
function main(params, callback){
var result = [
{
"key": "temperature",
"value": params.payload.payload_fields.temperature
},
{
"key": "$settings.name",
"value": params.payload.hardware_serial
},
{
"key": "$geo",
"value": [params.payload.metadata.longitude,params.payload.metadata.latitude]
},
];
callback(null, result);
}
{
"app_id": "my-app-id", // Same as in the topic
"dev_id": "my-dev-id", // Same as in the topic
"hardware_serial": "0102030405060708", // In case of LoRaWAN: the DevEUI
"port": 1, // LoRaWAN FPort
"counter": 2, // LoRaWAN frame counter
"is_retry": false, // Is set to true if this message is a retry (you could also detect this from the counter)
"confirmed": false, // Is set to true if this message was a confirmed message
"payload_raw": "AQIDBA==", // Base64 encoded payload: [0x01, 0x02, 0x03, 0x04]
"payload_fields": {"temperature": 25}, // Object containing the results from the payload functions - left out when empty
"metadata": {
"time": "1970-01-01T00:00:00Z", // Time when the server received the message
"frequency": 868.1, // Frequency at which the message was sent
"modulation": "LORA", // Modulation that was used - LORA or FSK
"data_rate": "SF7BW125", // Data rate that was used - if LORA modulation
"bit_rate": 50000, // Bit rate that was used - if FSK modulation
"coding_rate": "4/5", // Coding rate that was used
"gateways": [
{
"gtw_id": "ttn-herengracht-ams", // EUI of the gateway
"timestamp": 12345, // Timestamp when the gateway received the message
"time": "1970-01-01T00:00:00Z", // Time when the gateway received the message - left out when gateway does not have synchronized time
"channel": 0, // Channel where the gateway received the message
"rssi": -25, // Signal strength of the received message
"snr": 5, // Signal to noise ratio of the received message
"rf_chain": 0, // RF chain where the gateway received the message
"latitude": 52.1234, // Latitude of the gateway reported in its status updates
"longitude": 6.1234, // Longitude of the gateway
"altitude": 6 // Altitude of the gateway
},
//...more if received by more gateways...
],
"latitude": 52.2345, // Latitude of the device
"longitude": 6.2345, // Longitude of the device
"altitude": 2 // Altitude of the device
},
"downlink_url": "https://integrations.thethingsnetwork.org/ttn-eu/api/v2/down/my-app-id/my-process-id?key=ttn-account-v2.secret"
}
Once the URL is configured and the Cloud Code parser is coded, everything is ready to start receiving and monitoring your data.
Updated almost 2 years ago